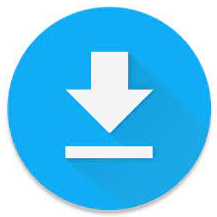
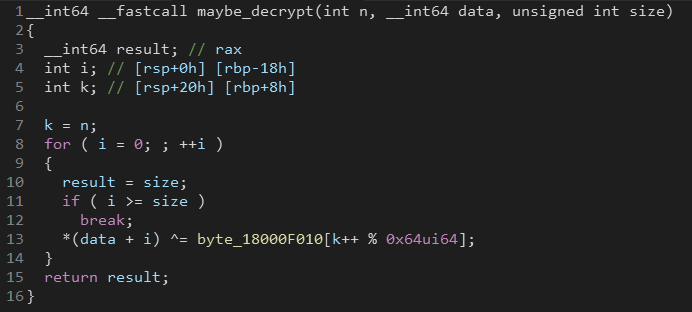
SelectComboboxItem(libCombo, selectItemIndex) SelectItemIndex = random.randrange(len(items)) LibCombo = findControl(findTopWindow(wantedText="ACE"), findAceLibrarySelectionFunction) # Find the ACE window library selection comboĭef findAceLibrarySelectionFunction(hwnd, windowText, windowClass): return windowClass = "ComboBox" and ("EVO1" in getComboboxItems(hwnd)) If anyone can suggest a more elegant way of doing this, I'd be pleased to hear of it. Unfortunately, it was not to be - while the selected item did change in the GUI, the application that I was automating didn't respond to the change without the click, and the drop-down list didn't disappear without the keystroke. I had hoped that the first of these messages would be enough. Win32gui.SendMessage(hwnd, win32con.WM_KEYUP, key, 0) Win32gui.SendMessage(hwnd, win32con.WM_KEYDOWN, key, 0) Win32gui.SendMessage(hwnd, win32con.CB_SETCURSEL, item, 0)

Last thing today - selection a combo-box item. ValueLength = win32gui.SendMessage(hwnd, getValueMessage, itemIndex, value)ĭef getComboboxItems(hwnd): return getMultipleWindowValues(hwnd, getCountMessage=win32con.CB_GETCOUNT, getValueMessage=win32con.CB_GETLBTEXT)ĭef getEditText(hwnd): return getMultipleWindowValues(hwnd, getCountMessage=win32con.EM_GETLINECOUNT, getValueMessage=win32con.EM_GETLINE) Looks a bit like getEditText, doesn't it? I thought so - refactored version below:ĭef getMultipleWindowValues(hwnd, getCountMessage, getValueMessage):Ĭount = win32gui.SendMessage(hwnd, getCountMessage, 0, 0) Linelength = win32gui.SendMessage(hwnd, win32con.CB_GETLBTEXT, itemIndex, linetext) ItemCount = win32gui.SendMessage(hwnd, win32con.CB_GETCOUNT, 0, 0)
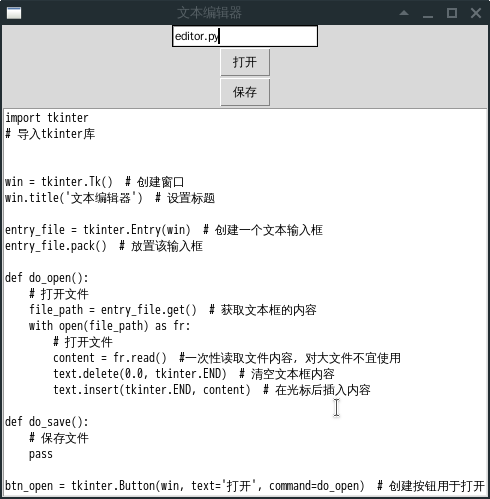
GETWINDOWTEXT PYTHON CODE
(These are the ones with a little down-arrow button to the right hand side, allowing you to select one (or perhaps more) from several options.) Here is the code to get a list of the values from a combo box: GenEdit = findControl(genTop, editFinder) GenTop = findTopWindow(wantedText="Generating the Runtime")ĭef editFinder(hwnd, windowText, windowClass): return windowClass = "Edit" Or perhaps it should be a generator? Anyway, as it stands, you can use it like so: struct to the rescue! It would be nice to wrap this up into a class, implementing a file-like interface, at some point. The first byte of this string must contain the total length of the string. When sending the win32con.EM_GETLINE, you need to pass a string, into which the line of text will be inserted. The only complicated bit here is the struct stuff. Linelength = win32gui.SendMessage(hwnd, win32con.EM_GETLINE, line, linetext) Linecount = win32gui.SendMessage(hwnd, win32con.EM_GETLINECOUNT, 0, 0) Here's a function which will get it for you: An edit control is one of these - it has lines of text rather than just text. But other classes don't have the kind of text that win32gui.GetWindowText() sets. The thing is, this works for some classes of window - you can use win32gui.GetWindowText() to get the text of a button or a label, for example. "But wait", you'll say, "can't you use the win32gui.GetWindowText() function"? No, actually, you can't.

I prefer the first form, but that's purely subjective.Īnd now, for my next trick, let's get the text from an Edit control. OkButton = findControl(optDialog, lambda hwnd, windowText, windowClass : windowClass = "Button" and windowText = "OK") You could do this more tersely with a lambda, by the way: OkButton = findControl(optDialog, findAButtonCalledOK) Return windowClass = "Button" and windowText = "OK" OptDialog = findTopWindow(wantedText="Options")ĭef findAButtonCalledOK(hwnd, windowText, windowClass): # Define a function to find our button Win32gui.SendMessage(hwnd, win32con.WM_LBUTTONUP, 0, 0) Win32gui.SendMessage(hwnd, win32con.WM_LBUTTONDOWN, 0, 0) I'll just give a few simple examples, from which you should be able to extrapolate most things that you might need to do.Ī great many of these actions are class specific - you can select one or many of a combo box's items, for example, but a button doesn't have items to select. Anything that you can do with a mouse or a keyboard can be automated. There are, of course, loads of things you can do with a control. See Driving win32 GUIs with Python, part 1 and Driving win32 GUIs with Python, part 2. Some things you can do with a control once you have it.
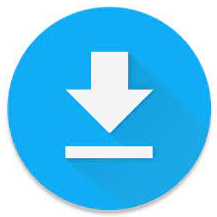